How to get dominant colors of an image in PHP
Table of contents:
- # Introduction
- # Getting the dominant colors of an image
- # Where I use the dominant color of an image
# Introduction
Web design seems to be a thing for graphic designers, but it is also important for web developers. One way to make a website look good is to use colors that match the content of the website.
One of this thing is creating user profiles. Setting a header/banner on a user profile is a common thing to do on social media platforms. Instead of asking the user to upload a separate banner, we can use the dominant color of the profile picture as the background color of the header.
In this article, we will see how to get the dominant colors of an image in PHP.
# Getting the dominant colors of an image
First, we need to install the thephpleague/color-extractor
package. You can install the package via composer using the following command:
composer require league/color-extractor
To get the dominant colors of an image, we will use the League\ColorExtractor\Palette
class provided by the package.
The Palette
class has a static method fromUrl
that takes the URL of the image as an argument. Other methods are fromFilename
, fromContents
and fromGD
:
use League\ColorExtractor\Palette;
$palette = Palette::fromUrl('https://courseants.com/images/logo.png');
We can then use the getMostUsedColors
method to get the dominant colors of the image. As an argument, we can pass the number of dominant colors we want to get:
$colors = $palette->getMostUsedColors(5);
Colors are returned as an integer in key-value pairs, where the key is the color and the value is the number of times the color is used in the image:
[
13995833 => 7713,
2301728 => 6483,
15510080 => 1722,
14785853 => 1537,
13996089 => 1446,
]
To use our colors in the frontend, we should convert the integer of one of the colors to hex format using the League\ColorExtractor\Color
class:
use League\ColorExtractor\Color;
Color::fromIntToHex(array_key_first($colors)); // #D58F39
# Full example
use League\ColorExtractor\Palette;
use League\ColorExtractor\Color;
class HomeViewModel extends ViewModel
{
public function color(): string
{
$palette = Palette::fromUrl('https://courseants.com/images/logo.png');
$colors = $palette->getMostUsedColors(5);
return Color::fromIntToHex(array_key_first($colors));
}
}
As we have the dominant color of the image in hex format, we can use it as the background color of a div in our frontend. In a Blade file, we can do the following:
<div class="relative">
<div class="h-32 w-full lg:h-48" style="background-color: {{ $color }};">
</div>
</div>
# Where I use the dominant color of an image
I use the dominant color as the background color of the platform profile header on Courseants. You can see an example of this on the Spatie platform page:
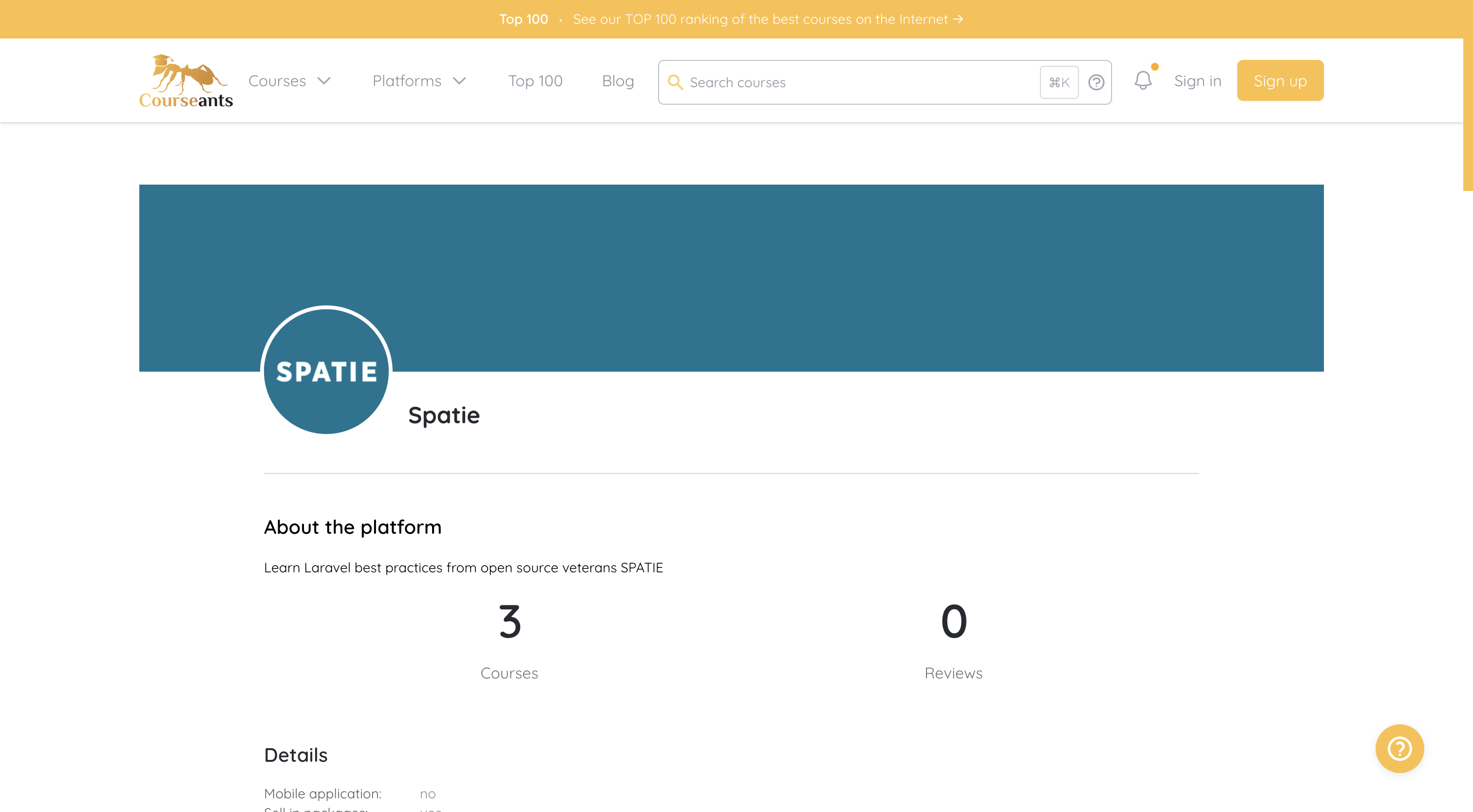